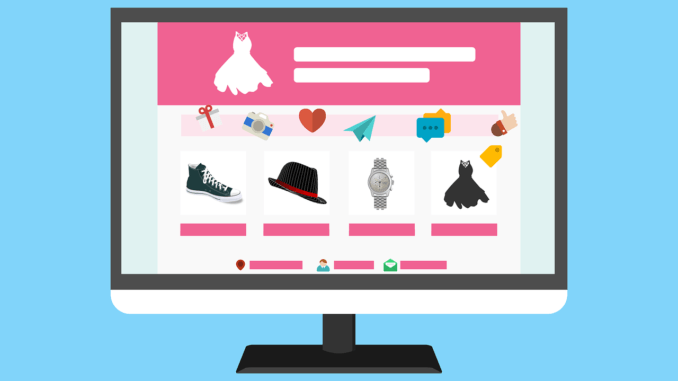
Why do you need to add additional fields to WooCommerce categories
Every once in a while the built in fields won’t be enough and you’ll have to add your own in order to adjust a website for your needs. In my particular case I needed to add additional description for after the main content in a category page and this is how I accomplished that
- Figure out which hooks you need to add your actions too
- Add your fields
- Add the “Save” action
- Use our new field
Figure out which hooks you need to add your actions too
After some research I came accross the “{taxonomy}_add_form_fields” and “{taxonomy}_edit_form_fields” in the WordPress developer resources. Digging a little more I figured out that the categories taxonomy is called “product_cat”. Now that we know where to add our fields, we need to figure out how to save the data. A little digging later I found “edited_{taxonomy}” and “create_{taxonomy}”. Now lets code!
Add your fields
Add your field to the “Add category” screen
Now when we’ve done the initial research, adding the fields is simply a matter of outputting the HTML
add_action('product_cat_add_form_fields', function() { ?> <div class="form-field"> <label for="second_description"><?php _e('Second description', 'wh'); ?></label> <?php wp_editor( '', 'second_description', ['textarea_name' => 'second_description'] ); ?> </div> <?php }, 10, 1);
Add your field to the “Edit category” screen
Adding the field to the “Edit” screen is sort of similar to the “Add” screen but we have to retrieve the initial value first, because there might already be a saved value.
add_action('product_cat_edit_form_fields', function($term) { //getting term ID $term_id = $term->term_id; // retrieve the existing value(s) for this meta field. $second_description = get_term_meta($term_id, 'second_description', true); ?> <tr class="form-field"> <th scope="row" valign="top"><label for="second_description"><?php _e('Second description', 'wh'); ?></label></th> <td> <?php wp_editor( $second_description, 'second_description', ['textarea_name' => 'second_description'] ); ?> </td> </tr> <?php }, 10, 1);
Add the “Save” action
Now we need to handle the saving of our data from the both “Add” and “Edit” screens:
add_action('edited_product_cat', 'wb_save_taxonomy_custom_meta', 10, 1); add_action('create_product_cat', 'wb_save_taxonomy_custom_meta', 10, 1); // Save extra taxonomy fields callback function. function wb_save_taxonomy_custom_meta($term_id) { $second_description = filter_input(INPUT_POST, 'second_description'); update_term_meta($term_id, 'second_description', $second_description); }
Use our new field
Now in my case I wanted to add the text from the field just below the main content in the category page, and the hook ‘woocommerce_after_main_content’ was exactly what I needed
add_action( 'woocommerce_after_main_content', function(){ if ( is_tax( array( 'product_cat', 'product_tag' ) ) && 0 === absint( get_query_var( 'paged' ) ) ) { $term = get_queried_object(); $term_id = $term->term_id; $second_description = get_term_meta($term_id, 'second_description', true); if ( $second_description ) { echo '<div class="term-description">' . $second_description . '</div>'; } } }, 9 );
And voila this is my thought process behind adding additional fields to custom taxonomies and more precisely product_cat in WooCommerce
You can download the code for this how-to in