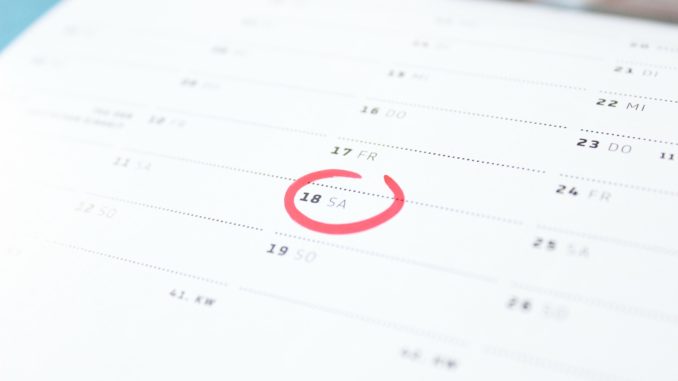
Many people are having trouble comparing dates with PHP, so I decided to introduce one function which makes the process very easy. The function is called strtotime and it is used to convert regular dates(Ex.: 2012-08-26 20:26 ) to Unix/POSIX time. You can check the definition of Unix/POSIX time in WikiPedia.
Now lets see the code:
echo strtotime("2012-08-26 20:26"); //returns 1346001960
In order to get the current timestamp you can use:
echo strtotime('now');
The power of strtotime, comes from the fact that it recognizes some keywords:
echo strtotime("now"), "n"; echo strtotime("24 August 2012"), "n"; echo strtotime("+1 day"), "n"; echo strtotime("+1 week"), "n"; echo strtotime("+1 week 2 days 4 hours 2 seconds"), "n"; echo strtotime("next Friday"), "n"; echo strtotime("last Sunday"), "n";
Now, the interesting(and relevant) part…comparing dates.
// First date $dateBefore = '2012-08-01 12:38'; // Second date $dateAfter = '2012-08-24 15:23'; if(strtotime($dateAfter) > strtotime($dateBefore)){ // your code goes here }
That is pretty much it…you can now use the function to build date filters and any kind of functionality involving dates.
Leave a Reply