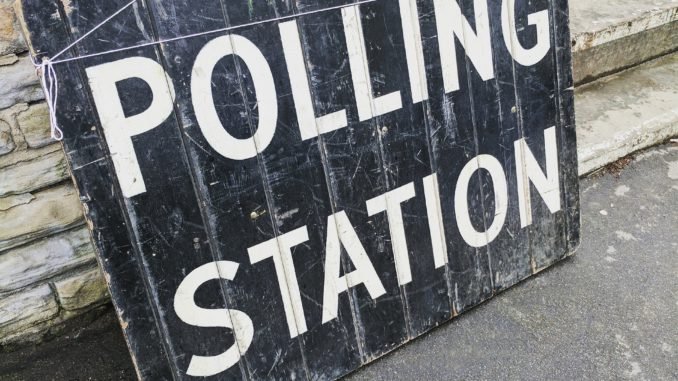
What is Polling?
The web (or HTTP 1.1 to be correct) is build on top of request – response cycle, in this way of communication though the server can’t send updates to the client when data changes, unless the client makes a request. “Polling” is the action of periodically requesting a given resource to check for changes. Very handy in reactive apps, where we want to seamlessly update the data.
How to do polling in Vue.JS?
I’ll give you a very simple implementation of polling in Vue.JS which I’m using all the time and it seems to be working flawlessly so far.
new Vue({ el: '#pollingExample', data: { discountedProducts: [] }, methods: { loadData: function () { $.get('/store/discounts', function (response) { this.discountedProducts = response.discountedProducts; }.bind(this)); } }, mounted: function () { //Initial Load this.loadData(); //Run every 30 seconds var loadData = function(){ this.loadData(); // Do stuff setTimeout(loadData, 30000); }; setTimeout(loadData,30000); } }); <div id="pollingExample"> <div v-for="product in discountedProducts">{{ product.name + "-" +product.price + "$" }}</div> </div>
There is nothing special in the code above, I simply create a function that loads the data(loadData), and the more interesting part is hooking into the mounted function, which is a built in method called right after the instance has been mounted. That is our magic bullet right there, when the instance is mounted we load the data initially and with the help of setInterval we schedule our function to run every 30 seconds.
This is how you do polling in Vue.JS with just a few simple steps.
In javascript, is an accepted fact to avoid the use of setInterval in favor of the setTimeout. The reason is that if you set an short interval and your action needs more than the interval to complete, you will end up having a bottleneck. It is recommended to use setTimeout when the action is completed in order to schedule a new call of the method.
http://reallifejs.com/brainchunks/repeated-events-timeout-or-interval/
You are indeed correct. I’ll update the article.
the timer should be cleaned before component destruction to avoid memory leakp