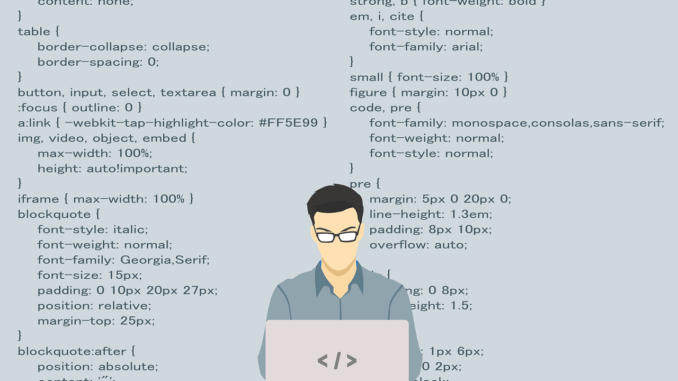
Dealing with forms in WordPress plugins sooner or later you’ll have to redirect the user on successful or failed submission. An example situation is that a user submits a form in your website, and you want to return some feedback based on the form data, eg “Fill more data” or “Successful submission”. One way to achieve that is to serialize the data and save it into Cookie, however if user does not accept cookies your data will not pass through. The solution I like is saving the data I want to pass in a transient and returning the id as a GET parameter, in that way you’re sure that the information will get delivered and WordPress will handle serialization/unserialization if you store objects or arrays in the transient. Now a little code:
//functions.php function generateRandomString($length = 5) { $characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'; $charactersLength = strlen($characters); $randomString = ''; for ($i = 0; $i < $length; ++$i) { $randomString .= $characters[rand(0, $charactersLength - 1)]; } return $randomString; } add_action('init', function() { if (isset($_POST["submission"])) { $validation = function() { $errors = []; //Validate //... return $errors; }; if(empty($validation())) { $signature = generateRandomString(); $response = [ 'success' => true, 'message' => 'Thanks for your submission', ]; set_transient($signature, $response, 10 * MINUTE_IN_SECONDS); global $wp; wp_redirect(add_query_arg('fid', $signature, home_url($wp->request).$_SERVER['REQUEST_URI'])); exit; } } });
In this example after your form validation you want to return your feedback to the user and you’re doing that by:
- Create a signature from a random string of 5 characters
- Create a transient with that signature as a name and your object/array as value, setting the expiration for 10 minutes
- Using wp_redirect to redirect the user to the same url he came from but using add_query_arg you pass your signature as a GET parameter to the url
How to use that information in your template is fairly simple:
//Your template <?php if (isset($_GET['fid'])) { $notification = get_transient($_GET['fid']); ?> //Do something with the notification echo $notification['message']; }
This is how you redirect user after form submission in WordPress and how to pass feedback from init hook to the front-end.
You can download the code for this how-to in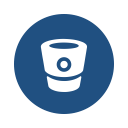
Leave a Reply